Hello, esteemed Habr contributors!
Introduction
In modern web development, the boundaries between classic and web applications are blurring every day. Today we can create not only interactive websites, but also full-fledged games right in the browser. One of the tools that makes this possible is the React Three Fiber library - a powerful tool for creating 3D graphics based on Three.js using React technology.
About the React Three Fiber stack
React Three Fiber is a wrapper over Three.js that uses the structure and principles of React to create 3D graphics on the web. This stack allows developers to combine the power of Three.js with the convenience and flexibility of React, making the process of creating an application more intuitive and organised.
At the heart of React Three Fiber is the idea that everything you create in a scene is a React component. This allows developers to apply familiar patterns and methodologies.
One of the main advantages of React Three Fiber is its ease of integration with the React ecosystem. Any other React tools can still be easily integrated when using this library.
Relevance of Web-GameDev
Web-GameDev has undergone major changes in recent years, evolving from simple 2D Flash games to complex 3D projects comparable to desktop applications. This growth in popularity and capabilities makes Web-GameDev an area that cannot be ignored.
One of the main advantages of web gaming is its accessibility. Players do not need to download and install any additional software - just click on the link in their browser. This simplifies the distribution and promotion of games, making them available to a wide audience around the world.
Finally, web game development can be a great way for developers to try their hand at gamedev using familiar technologies. Thanks to the available tools and libraries, even without experience in 3D graphics, it is possible to create interesting and high-quality projects!
Game performance in modern browsers
Modern browsers have come a long way, evolving from fairly simple web browsing tools to powerful platforms for running complex applications and games. Major browsers such as Chrome, Firefox, Edge and others are constantly being optimised and developed to ensure high performance, making them an ideal platform for developing complex applications.
One of the key tools that has fuelled the development of browser-based gaming is WebGL. This standard allowed developers to use hardware graphics acceleration, which significantly improved the performance of 3D games. Together with other webAPIs, WebGL opens up new possibilities for creating impressive web applications directly in the browser.
Nevertheless, when developing games for the browser, it is crucial to consider various performance aspects: resource optimisation, memory management and adaptation for different devices are all key points that can affect the success of a project.
On your mark!
However, words and theory are one thing, but practical experience is quite another. To really understand and appreciate the full potential of web game development, the best way is to immerse yourself in the development process. Therefore, as an example of successful web game development, we will create our own game. This process will allow us to learn key aspects of development, face real problems and find solutions to them, and see how powerful and flexible a web game development platform can be.
In a series of articles, we'll look at how to create a first-person shooter using the features of this library, and dive into the exciting world of web-gamedev!
Final demo
Repository on GitHub
Now, let's get started!
Setting up the project and installing packages
First of all, we will need a React project template. So let's start by installing it.
npm create vite@latest
select the React library;
select JavaScript.
Install additional npm packages.
npm install three @react-three/fiber @react-three/drei @react-three/rapier zustand @tweenjs/tween.js
Then delete everything unnecessary from our project.
Customising the Canvas display
In the main.jsx file, add a div element that will be displayed on the page as a scope. Insert a Canvas component and set the field of view of the camera. Inside the Canvas component place the App component.

main.jsx
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App.jsx'
import './index.css'
import {Canvas} from "@react-three/fiber";
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<div id="container">
<div className="aim"></div>
<Canvas camera={{ fov: 45 }}>
<App />
</Canvas>
</div>
</React.StrictMode>,
)
Let's add styles to stretch the UI elements to the full height of the screen and display the scope as a circle in the centre of the screen.

index.css
* {
margin: 0;
padding: 0;
}
html, body, #root, #container {
height: 100%;
}
.aim {
position: absolute;
top: 50%;
left: 50%;
width: 10px;
height: 10px;
border-radius: 50%;
transform: translate3d(-50%, -50%, 0);
border: 2px solid white;
z-index: 2;
}
In the App component we add a Sky component, which will be displayed as the background in our game scene in the form of a sky.

App.jsx
import {Sky} from "@react-three/drei";
export const App = () => {
return (
<>
<Sky sunPosition={[100, 20, 100]} />
</>
)
}
export default App

Floor surface
Let's create a Ground component and place it in the App component.

App.jsx
import {Sky} from "@react-three/drei";
import {Ground} from "./Ground.jsx";
export const App = () => {
return (
<>
<Sky sunPosition={[100, 20, 100]}/>
<Ground />
</>
)
}
export default App
In Ground, create a flat surface element. On the Y axis move it downwards so that this plane is in the field of view of the camera. And also flip the plane on the X axis to make it horizontal.

Ground.jsx
export const Ground = () => {
return (
<mesh position={[0, -5, 0]} rotation-x={-Math.PI / 2}>
<planeGeometry args={[500, 500]} />
<meshStandardMaterial color="gray" />
</mesh>
);
}
Even though we specified grey as the material colour, the plane appears completely black.

Basic lighting
By default, there is no lighting in the scene, so let's add a light source ambientLight, which illuminates the object from all sides and does not have a directed beam. As a parameter set the intensity of the glow.

App.jsx
import {Sky} from "@react-three/drei";
import {Ground} from "./Ground.jsx";
export const App = () => {
return (
<>
<Sky sunPosition={[100, 20, 100]}/>
<ambientLight intensity={1.5} />
<Ground />
</>
)
}
export default App

Texture for the floor surface
To make the floor surface not look homogeneous, we will add texture. Make a pattern of the floor surface in the form of cells repeating all along the surface.
In the assets folder add a PNG image with a texture.

To load a texture on the scene, let's use the useTexture hook from the @react-three/drei package. And as a parameter for the hook we will pass the texture image imported into the file. Set the repetition of the image in the horizontal axes.
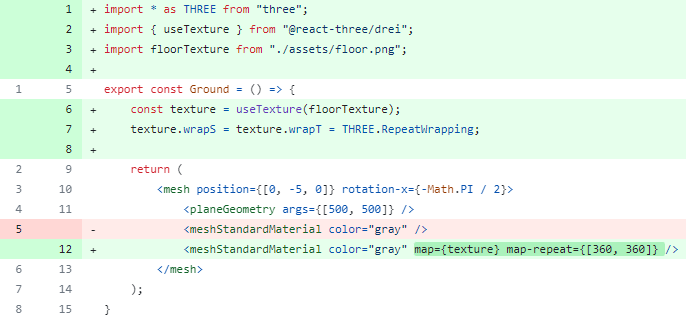
Ground.jsx
import * as THREE from "three";
import { useTexture } from "@react-three/drei";
import floorTexture from "./assets/floor.png";
export const Ground = () => {
const texture = useTexture(floorTexture);
texture.wrapS = texture.wrapT = THREE.RepeatWrapping;
return (
<mesh position={[0, -5, 0]} rotation-x={-Math.PI / 2}>
<planeGeometry args={[500, 500]} />
<meshStandardMaterial color="gray" map={texture} map-repeat={[360, 360]} />
</mesh>
);
}
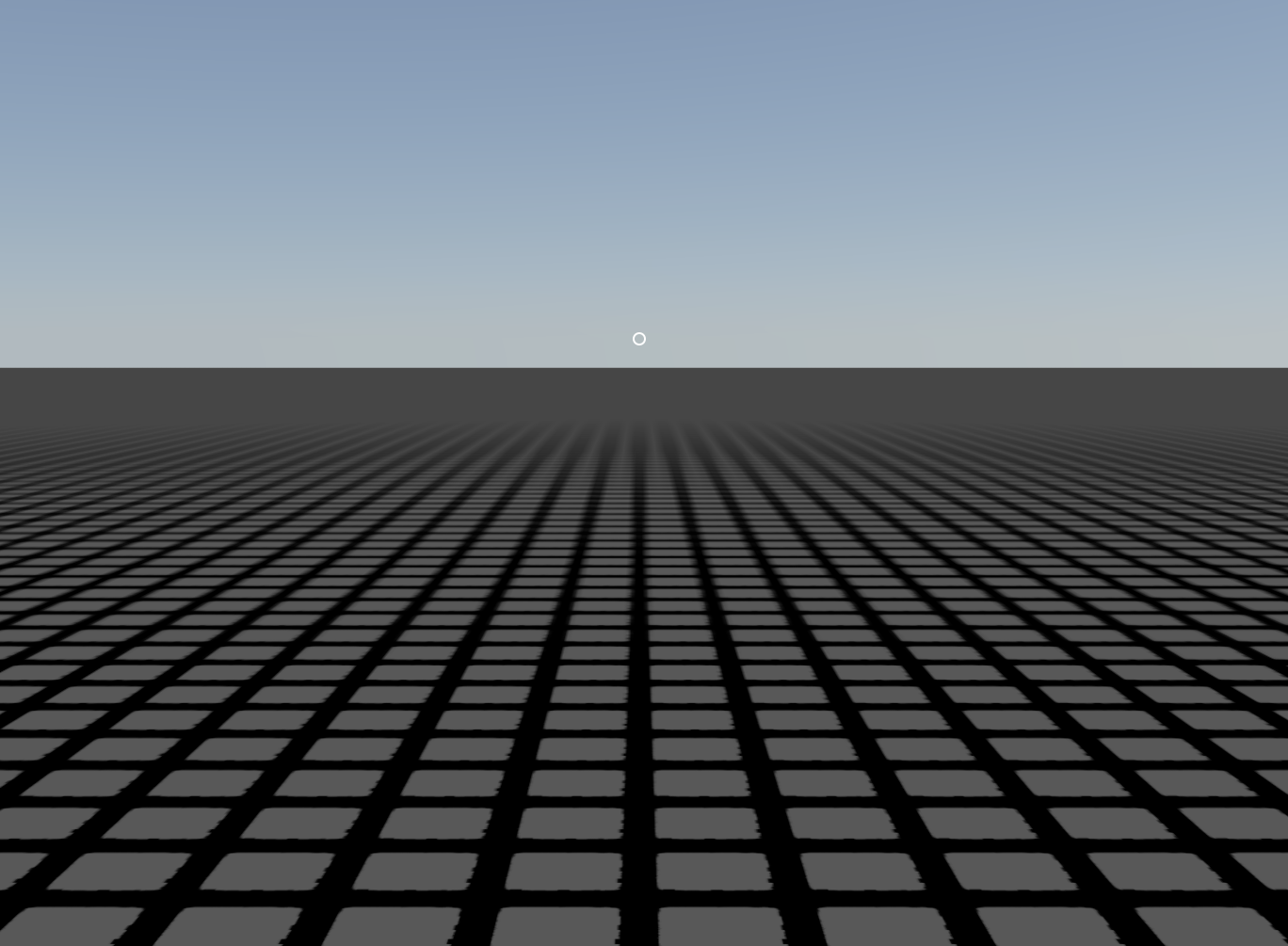
Camera movement
Using the PointerLockControls component from the @react-three/drei package, fix the cursor on the screen so that it does not move when you move the mouse, but changes the position of the camera on the scene.

App.jsx
import {PointerLockControls, Sky} from "@react-three/drei";
import {Ground} from "./Ground.jsx";
export const App = () => {
return (
<>
<PointerLockControls />
<Sky sunPosition={[100, 20, 100]}/>
<ambientLight intensity={1.5} />
<Ground />
</>
)
}
export default App
Let's make a small edit for the Ground component.

Ground.jsx
import * as THREE from "three";
import { useTexture } from "@react-three/drei";
import floorTexture from "./assets/floor.png";
export const Ground = () => {
const texture = useTexture(floorTexture);
texture.wrapS = texture.wrapT = THREE.RepeatWrapping;
return (
<mesh position={[0, -5, 0]} rotation-x={-Math.PI / 2}>
<planeGeometry args={[500, 500]} />
<meshStandardMaterial color="gray" map={texture} map-repeat={[100, 100]} />
</mesh>
);
}
Adding physics
For clarity, let's add a simple cube to the scene.
<mesh position={[0, 3, -5]}>
<boxGeometry />
</mesh>

Right now he's just hanging in space.
Use the Physics component from the @react-three/rapier package to add "physics" to the scene. As a parameter, configure the gravity field, where we set the gravitational forces along the axes.
<Physics gravity={[0, -20, 0]}>
<Ground />
<mesh position={[0, 3, -5]}>
<boxGeometry />
</mesh>
</Physics>
However, our cube is inside the physics component, but nothing happens to it. To make the cube behave like a real physical object, we need to wrap it in the RigidBody component from the @react-three/rapier package.

App.jsx
import {PointerLockControls, Sky} from "@react-three/drei";
import {Ground} from "./Ground.jsx";
import {Physics, RigidBody} from "@react-three/rapier";
export const App = () => {
return (
<>
<PointerLockControls />
<Sky sunPosition={[100, 20, 100]}/>
<ambientLight intensity={1.5} />
<Physics gravity={[0, -20, 0]}>
<Ground />
<RigidBody>
<mesh position={[0, 3, -5]}>
<boxGeometry />
</mesh>
</RigidBody>
</Physics>
</>
)
}
export default App
After that, we will immediately see that every time the page reloads, the cube falls down under the influence of gravity.
But now there is another task - it is necessary to make the floor an object with which the cube can interact, and beyond which it will not fall.
The floor as a physical object
Let's go back to the Ground component and add a RigidBody component as a wrapper over the floor surface.

Ground.jsx
import * as THREE from "three";
import { useTexture } from "@react-three/drei";
import floorTexture from "./assets/floor.png";
import {RigidBody} from "@react-three/rapier";
export const Ground = () => {
const texture = useTexture(floorTexture);
texture.wrapS = texture.wrapT = THREE.RepeatWrapping;
return (
<RigidBody>
<mesh position={[0, -5, 0]} rotation-x={-Math.PI / 2}>
<planeGeometry args={[500, 500]} />
<meshStandardMaterial color="gray" map={texture} map-repeat={[100, 100]} />
</mesh>
</RigidBody>
);
}
Now when falling, the cube stays on the floor like a real physical object.
Subjecting a character to the laws of physics
Let's create a Player component that will control the character on the scene.
The character is the same physical object as the added cube, so it must interact with the floor surface as well as the cube on the scene. That's why we add the RigidBody component. And let's make the character in the form of a capsule.

Player.jsx
import {RigidBody} from "@react-three/rapier";
export const Player = () => {
return (
<>
<RigidBody position={[0, 1, -2]}>
<mesh>
<capsuleGeometry args={[0.5, 0.5]}/>
</mesh>
</RigidBody>
</>
);
}
Place the Player component inside the Physics component.

App.jsx
import {PointerLockControls, Sky} from "@react-three/drei";
import {Ground} from "./Ground.jsx";
import {Physics, RigidBody} from "@react-three/rapier";
import {Player} from "./Player.jsx";
export const App = () => {
return (
<>
<PointerLockControls />
<Sky sunPosition={[100, 20, 100]}/>
<ambientLight intensity={1.5} />
<Physics gravity={[0, -20, 0]}>
<Ground />
<Player />
<RigidBody>
<mesh position={[0, 3, -5]}>
<boxGeometry />
</mesh>
</RigidBody>
</Physics>
</>
)
}
export default App
Now our character has appeared on the scene.

Moving a character - creating a hook
The character will be controlled using the WASD keys, and jump using the Spacebar.
With our own react-hook, we implement the logic of moving the character.
Let's create a hooks.js file and add a new usePersonControls function there.
Let's define an object in the format {"keycode": "action to be performed"}. Next, add event handlers for pressing and releasing keyboard keys. When the handlers are triggered, we will determine the current actions being performed and update their active state. As a final result, the hook will return an object in the format {"action in progress": "status"}.

hooks.js
import { useEffect, useState } from "react";
export const usePersonControls = () => {
const keys = {
KeyW: "forward",
KeyS: "backward",
KeyA: "left",
KeyD: "right",
Space: "jump"
}
const moveFieldByKey = (key) => keys[key];
const [movement, setMovement] = useState({
forward: false,
backward: false,
left: false,
right: false,
jump: false
});
const setMovementStatus = (code, status) => {
setMovement((m) => ({...m, [code]: status}))
}
useEffect(() => {
const handleKeyDown = (ev) => {
setMovementStatus(moveFieldByKey(ev.code), true);
}
const handleKeyUp = (ev) => {
setMovementStatus(moveFieldByKey(ev.code), false);
}
document.addEventListener('keydown', handleKeyDown);
document.addEventListener('keyup', handleKeyUp);
return () => {
document.removeEventListener('keydown', handleKeyDown);
document.removeEventListener('keyup', handleKeyUp);
}
}, []);
return movement;
}
Moving a character - implementing a hook
After implementing the usePersonControls hook, it should be used when controlling the character. In the Player component we will add motion state tracking and update the vector of the character's movement direction.
We will also define variables that will store the states of the movement directions.

To update the character's position, let's useFrame provided by the @react-three/fiber package. This hook works similarly to requestAnimationFrame and executes the body of the function about 60 times per second.

Code Explanation:
const playerRef = useRef();
Create a link for the player object. This link will allow direct interaction with the player object on the scene.const { forward, backward, left, right, jump } = usePersonControls();
When a hook is used, an object with boolean values indicating which control buttons are currently pressed by the player is returned.useFrame((state) => { ... });
The hook is called on each frame of the animation. Inside this hook, the player's position and linear velocity are updated.if (!playerRef.current) return;
Checks for the presence of a player object. If there is no player object, the function will stop execution to avoid errors.const velocity = playerRef.current.linvel();
Get the current linear velocity of the player.frontVector.set(0, 0, backward - forward);
Set the forward/backward motion vector based on the pressed buttons.sideVector.set(left - right, 0, 0);
Set the left/right movement vector.direction.subVectors(frontVector, sideVector).normalize().multiplyScalar(MOVE_SPEED);
Calculate the final vector of player movement by subtracting the movement vectors, normalising the result (so that the vector length is 1) and multiplying by the movement speed constant.playerRef.current.wakeUp();
"Wakes up" the player object to make sure it reacts to changes. If you don't use this method, after some time the object will "sleep" and will not react to position changes.playerRef.current.setLinvel({ x: direction.x, y: velocity.y, z: direction.z });
Set the player's new linear velocity based on the calculated direction of movement and keep the current vertical velocity (so as not to affect jumps or falls).
Player.jsx
import * as THREE from "three";
import {RigidBody} from "@react-three/rapier";
import {useRef} from "react";
import {usePersonControls} from "./hooks.js";
import {useFrame} from "@react-three/fiber";
const MOVE_SPEED = 5;
const direction = new THREE.Vector3();
const frontVector = new THREE.Vector3();
const sideVector = new THREE.Vector3();
export const Player = () => {
const playerRef = useRef();
const { forward, backward, left, right, jump } = usePersonControls();
useFrame((state) => {
if (!playerRef.current) return;
const velocity = playerRef.current.linvel();
frontVector.set(0, 0, backward - forward);
sideVector.set(left - right, 0, 0);
direction.subVectors(frontVector, sideVector).normalize().multiplyScalar(MOVE_SPEED);
playerRef.current.wakeUp();
playerRef.current.setLinvel({ x: direction.x, y: velocity.y, z: direction.z });
});
return (
<>
<RigidBody position={[0, 1, -2]} ref={playerRef}>
<mesh>
<capsuleGeometry args={[0.5, 0.5]}/>
</mesh>
</RigidBody>
</>
);
}
As a result, when pressing the WASD keys, the character started moving around the scene. He can also interact with the cube, because they are both physical objects.
Moving a character - jump
In order to implement the jump, let's use the functionality from the @dimforge/rapier3d-compat and @react-three/rapier packages. In this example, let's check that the character is on the ground and the jump key has been pressed. In this case, we set the character's direction and acceleration force on the Y-axis.
For Player we will add mass and block rotation on all axes, so that he will not fall over in different directions when colliding with other objects on the scene.

Code Explanation:
const world = rapier.world;
Gaining access to the Rapier physics engine scene. It contains all physical objects and manages their interaction.const ray = world.castRay(new RAPIER.Ray(playerRef.current.translation(), { x: 0, y: -1, z: 0 }));
This is where "raycasting" (raycasting) takes place. A ray is created that starts at the player's current position and points down the y-axis. This ray is "cast" into the scene to determine if it intersects with any object in the scene.const grounded = ray && ray.collider && Math.abs(ray.toi) <= 1.5;
The condition is checked if the player is on the ground:
ray - whether the ray was created;
ray.collider - whether the ray collided with any object on the scene;
Math.abs(ray.toi) - the "exposure time" of the ray. If this value is less than or equal to the given value, it may indicate that the player is close enough to the surface to be considered "on the ground".
Player.jsx
import * as THREE from "three";
import * as RAPIER from "@dimforge/rapier3d-compat"
import {RigidBody, useRapier} from "@react-three/rapier";
import {useRef} from "react";
import {usePersonControls} from "./hooks.js";
import {useFrame} from "@react-three/fiber";
const MOVE_SPEED = 5;
const direction = new THREE.Vector3();
const frontVector = new THREE.Vector3();
const sideVector = new THREE.Vector3();
export const Player = () => {
const playerRef = useRef();
const { forward, backward, left, right, jump } = usePersonControls();
const rapier = useRapier();
useFrame((state) => {
if (!playerRef.current) return;
// moving player
const velocity = playerRef.current.linvel();
frontVector.set(0, 0, backward - forward);
sideVector.set(left - right, 0, 0);
direction.subVectors(frontVector, sideVector).normalize().multiplyScalar(MOVE_SPEED);
playerRef.current.wakeUp();
playerRef.current.setLinvel({ x: direction.x, y: velocity.y, z: direction.z });
// jumping
const world = rapier.world;
const ray = world.castRay(new RAPIER.Ray(playerRef.current.translation(), { x: 0, y: -1, z: 0 }));
const grounded = ray && ray.collider && Math.abs(ray.toi) <= 1;
if (jump && grounded) doJump();
});
const doJump = () => {
playerRef.current.setLinvel({x: 0, y: 8, z: 0});
}
return (
<>
<RigidBody position={[0, 1, -2]} mass={1} ref={playerRef} lockRotations>
<mesh>
<capsuleGeometry args={[0.5, 0.5]}/>
</mesh>
</RigidBody>
</>
);
}
You also need to modify the Ground component so that the raytraced algorithm for determining the "landing" status works correctly, by adding a physical object that will interact with other objects in the scene.

Ground.jsx
import * as THREE from "three";
import { useTexture } from "@react-three/drei";
import floorTexture from "./assets/floor.png";
import {CuboidCollider, RigidBody} from "@react-three/rapier";
export const Ground = () => {
const texture = useTexture(floorTexture);
texture.wrapS = texture.wrapT = THREE.RepeatWrapping;
return (
<RigidBody type="fixed" colliders={false}>
<mesh position={[0, 0, 0]} rotation-x={-Math.PI / 2}>
<planeGeometry args={[500, 500]} />
<meshStandardMaterial color="gray" map={texture} map-repeat={[100, 100]} />
</mesh>
<CuboidCollider args={[500, 2, 500]} position={[0, -2, 0]}/>
</RigidBody>
);
}
Let's raise the camera a little higher for a better view of the scene.

main.jsx
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App.jsx'
import './index.css'
import {Canvas} from "@react-three/fiber";
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<div id="container">
<div className="aim"></div>
<Canvas camera={{ fov: 45, position: [0, 5, 0] }}>
<App />
</Canvas>
</div>
</React.StrictMode>,
)

Section code
Moving the camera behind the character
To move the camera, we will get the current position of the player and change the position of the camera every time the frame is refreshed. And for the character to move exactly along the trajectory, where the camera is directed, we need to add applyEuler.

Code Explanation:
The applyEuler method applies rotation to a vector based on specified Euler angles. In this case, the camera rotation is applied to the direction vector. This is used to match the motion relative to the camera orientation, so that the player moves in the direction the camera is rotated.
Let's slightly adjust the size of Player and make it taller relative to the cube, increasing the size of CapsuleCollider and fixing the "jump" logic.

Section code
Generation of cubes
To make the scene not feel completely empty, let's add cube generation. In the json file, list the coordinates of each of the cubes and then display them on the scene. To do this, create a file cubes.json, in which we will list an array of coordinates.
[
[0, 0, -7],
[2, 0, -7],
[4, 0, -7],
[6, 0, -7],
[8, 0, -7],
[10, 0, -7]
]
In the Cube.jsx file, create a Cubes component, which will generate cubes in a loop. And Cube component will be directly generated object.
import {RigidBody} from "@react-three/rapier";
import cubes from "./cubes.json";
export const Cubes = () => {
return cubes.map((coords, index) => <Cube key={index} position={coords} />);
}
const Cube = (props) => {
return (
<RigidBody {...props}>
<mesh castShadow receiveShadow>
<meshStandardMaterial color="white" />
<boxGeometry />
</mesh>
</RigidBody>
);
}
Let's add the created Cubes component to the App component by deleting the previous single cube.

App.jsx
import {PointerLockControls, Sky} from "@react-three/drei";
import {Ground} from "./Ground.jsx";
import {Physics} from "@react-three/rapier";
import {Player} from "./Player.jsx";
import {Cubes} from "./Cube.jsx";
export const App = () => {
return (
<>
<PointerLockControls />
<Sky sunPosition={[100, 20, 100]}/>
<ambientLight intensity={1.5} />
<Physics gravity={[0, -20, 0]}>
<Ground />
<Player />
<Cubes />
</Physics>
</>
)
}
export default App
Importing the model into the project
Now let's add a 3D model to the scene. Let's add a weapon model for the character. Let's start by looking for a 3D model. For example, let's take this one.
Download the model in GLTF format and unpack the archive in the root of the project.
In order to get the format we need to import the model into the scene, we will need to install the gltf-pipeline add-on package.
npm i -D gltf-pipeline
Using the gltf-pipeline package, reconvert the model from the GLTF format to the GLB format, since in this format all model data are placed in one file. As an output directory for the generated file we specify the public folder.
gltf-pipeline -i weapon/scene.gltf -o public/weapon.glb
Then we need to generate a react component that will contain the markup of this model to add it to the scene. Let's use the official resource from the @react-three/fiber developers.
Going to the converter will require you to load the converted weapon.glb file.
Using drag and drop or Explorer search, find this file and download it.

In the converter we will see the generated react-component, the code of which we will transfer to our project in a new file WeaponModel.jsx, changing the name of the component to the same name as the file.
Displaying the weapon model on the scene
Now let's import the created model to the scene. In App.jsx file add WeaponModel component.

App.jsx
import {PointerLockControls, Sky} from "@react-three/drei";
import {Ground} from "./Ground.jsx";
import {Physics} from "@react-three/rapier";
import {Player} from "./Player.jsx";
import {Cubes} from "./Cube.jsx";
import {WeaponModel} from "./WeaponModel.jsx";
export const App = () => {
return (
<>
<PointerLockControls />
<Sky sunPosition={[100, 20, 100]}/>
<ambientLight intensity={1.5} />
<Physics gravity={[0, -20, 0]}>
<Ground />
<Player />
<Cubes />
</Physics>
<group position={[0, 3, 0]}>
<WeaponModel />
</group>
</>
)
}
export default App
Adding shadows
At this point in our scene, none of the objects are casting shadows.
To enable shadows on the scene you need to add the shadows attribute to the Canvas component.

main.jsx
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App.jsx'
import './index.css'
import {Canvas} from "@react-three/fiber";
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<div id="container">
<div className="aim"></div>
<Canvas camera={{ fov: 45 }} shadows>
<App />
</Canvas>
</div>
</React.StrictMode>,
)
Next, we need to add a new light source. Despite the fact that we already have ambientLight on the scene, it cannot create shadows for objects, because it does not have a directional light beam. So let's add a new light source called directionalLight and configure it. The attribute to enable the "cast" shadow mode is castShadow. It is the addition of this parameter that indicates that this object can cast a shadow on other objects.

App.jsx
import {PointerLockControls, Sky} from "@react-three/drei";
import {Ground} from "./Ground.jsx";
import {Physics} from "@react-three/rapier";
import {Player} from "./Player.jsx";
import {Cubes} from "./Cube.jsx";
import {WeaponModel} from "./WeaponModel.jsx";
export const App = () => {
return (
<>
<PointerLockControls />
<Sky sunPosition={[100, 20, 100]}/>
<ambientLight intensity={1.5} />
<directionalLight
castShadow
intensity={.8}
position={[50, 50, 0]} />
<Physics gravity={[0, -20, 0]}>
<Ground />
<Player />
<Cubes />
</Physics>
<group position={[3, 1, -2]}>
<WeaponModel />
</group>
</>
)
}
export default App
After that, let's add another attribute receiveShadow to the Ground component, which means that the component in the scene can receive and display shadows on itself.

Ground.jsx
import * as THREE from "three";
import { useTexture } from "@react-three/drei";
import floorTexture from "./assets/floor.png";
import {CuboidCollider, RigidBody} from "@react-three/rapier";
export const Ground = () => {
const texture = useTexture(floorTexture);
texture.wrapS = texture.wrapT = THREE.RepeatWrapping;
return (
<RigidBody type="fixed" colliders={false}>
<mesh receiveShadow position={[0, 0, 0]} rotation-x={-Math.PI / 2}>
<planeGeometry args={[500, 500]} />
<meshStandardMaterial color="gray" map={texture} map-repeat={[100, 100]} />
</mesh>
<CuboidCollider args={[500, 2, 500]} position={[0, -2, 0]}/>
</RigidBody>
);
}

Similar attributes should be added to other objects on the scene: cubes and player. For the cubes we will add castShadow and receiveShadow, because they can both cast and receive shadows, and for the player we will add only castShadow.
Let's add castShadow for Player.

Player.jsx
import * as THREE from "three";
import * as RAPIER from "@dimforge/rapier3d-compat"
import {CapsuleCollider, RigidBody, useRapier} from "@react-three/rapier";
import {useRef} from "react";
import {usePersonControls} from "./hooks.js";
import {useFrame} from "@react-three/fiber";
const MOVE_SPEED = 5;
const direction = new THREE.Vector3();
const frontVector = new THREE.Vector3();
const sideVector = new THREE.Vector3();
export const Player = () => {
const playerRef = useRef();
const { forward, backward, left, right, jump } = usePersonControls();
const rapier = useRapier();
useFrame((state) => {
if (!playerRef.current) return;
// moving player
const velocity = playerRef.current.linvel();
frontVector.set(0, 0, backward - forward);
sideVector.set(left - right, 0, 0);
direction.subVectors(frontVector, sideVector).normalize().multiplyScalar(MOVE_SPEED).applyEuler(state.camera.rotation);
playerRef.current.wakeUp();
playerRef.current.setLinvel({ x: direction.x, y: velocity.y, z: direction.z });
// jumping
const world = rapier.world;
const ray = world.castRay(new RAPIER.Ray(playerRef.current.translation(), { x: 0, y: -1, z: 0 }));
const grounded = ray && ray.collider && Math.abs(ray.toi) <= 1.5;
if (jump && grounded) doJump();
// moving camera
const {x, y, z} = playerRef.current.translation();
state.camera.position.set(x, y, z);
});
const doJump = () => {
playerRef.current.setLinvel({x: 0, y: 8, z: 0});
}
return (
<>
<RigidBody colliders={false} mass={1} ref={playerRef} lockRotations>
<mesh castShadow>
<capsuleGeometry args={[0.5, 0.5]}/>
<CapsuleCollider args={[0.75, 0.5]} />
</mesh>
</RigidBody>
</>
);
}
Add castShadow and receiveShadow for Cube.

Cube.jsx
import {RigidBody} from "@react-three/rapier";
import cubes from "./cubes.json";
export const Cubes = () => {
return cubes.map((coords, index) => <Cube key={index} position={coords} />);
}
const Cube = (props) => {
return (
<RigidBody {...props}>
<mesh castShadow receiveShadow>
<meshStandardMaterial color="white" />
<boxGeometry />
</mesh>
</RigidBody>
);
}
Adding shadows - correcting shadow clipping
If you look closely now, you will find that the surface area on which the shadow is cast is quite small. And when going beyond this area, the shadow is simply cut off.
The reason for this is that by default the camera captures only a small area of the displayed shadows from directionalLight. We can for the directionalLight component by adding additional attributes shadow-camera-(top, bottom, left, right) to expand this area of visibility. After adding these attributes, the shadow will become slightly blurred. To improve the quality, we will add the shadow-mapSize attribute.

App.jsx
import {PointerLockControls, Sky} from "@react-three/drei";
import {Ground} from "./Ground.jsx";
import {Physics} from "@react-three/rapier";
import {Player} from "./Player.jsx";
import {Cubes} from "./Cube.jsx";
import {WeaponModel} from "./WeaponModel.jsx";
const shadowOffset = 50;
export const App = () => {
return (
<>
<PointerLockControls />
<Sky sunPosition={[100, 20, 100]}/>
<ambientLight intensity={1.5} />
<directionalLight
castShadow
intensity={1.5}
shadow-mapSize={4096}
shadow-camera-top={shadowOffset}
shadow-camera-bottom={-shadowOffset}
shadow-camera-left={shadowOffset}
shadow-camera-right={-shadowOffset}
position={[100, 100, 0]}
/>
<Physics gravity={[0, -20, 0]}>
<Ground />
<Player />
<Cubes />
</Physics>
<group position={[3, 1, -2]}>
<WeaponModel />
</group>
</>
)
}
export default App
Binding weapons to a character
Now let's add first-person weapon display. Create a new Weapon component, which will contain the weapon behaviour logic and the 3D model itself.
import {WeaponModel} from "./WeaponModel.jsx";
export const Weapon = (props) => {
return (
<group {...props}>
<WeaponModel />
</group>
);
}
Let's place this component on the same level as the RigidBody of the character and in the useFrame hook we will set the position and rotation angle based on the position of the values from the camera.

Player.jsx
import * as THREE from "three";
import * as RAPIER from "@dimforge/rapier3d-compat"
import {CapsuleCollider, RigidBody, useRapier} from "@react-three/rapier";
import {useRef} from "react";
import {usePersonControls} from "./hooks.js";
import {useFrame} from "@react-three/fiber";
import {Weapon} from "./Weapon.jsx";
const MOVE_SPEED = 5;
const direction = new THREE.Vector3();
const frontVector = new THREE.Vector3();
const sideVector = new THREE.Vector3();
const rotation = new THREE.Vector3();
export const Player = () => {
const playerRef = useRef();
const { forward, backward, left, right, jump } = usePersonControls();
const objectInHandRef = useRef();
const rapier = useRapier();
useFrame((state) => {
if (!playerRef.current) return;
// moving player
const velocity = playerRef.current.linvel();
frontVector.set(0, 0, backward - forward);
sideVector.set(left - right, 0, 0);
direction.subVectors(frontVector, sideVector).normalize().multiplyScalar(MOVE_SPEED).applyEuler(state.camera.rotation);
playerRef.current.wakeUp();
playerRef.current.setLinvel({ x: direction.x, y: velocity.y, z: direction.z });
// jumping
const world = rapier.world;
const ray = world.castRay(new RAPIER.Ray(playerRef.current.translation(), { x: 0, y: -1, z: 0 }));
const grounded = ray && ray.collider && Math.abs(ray.toi) <= 1.5;
if (jump && grounded) doJump();
// moving camera
const {x, y, z} = playerRef.current.translation();
state.camera.position.set(x, y, z);
// moving object in hand for the player
objectInHandRef.current.rotation.copy(state.camera.rotation);
objectInHandRef.current.position.copy(state.camera.position).add(state.camera.getWorldDirection(rotation));
});
const doJump = () => {
playerRef.current.setLinvel({x: 0, y: 8, z: 0});
}
return (
<>
<RigidBody colliders={false} mass={1} ref={playerRef} lockRotations>
<mesh castShadow>
<capsuleGeometry args={[0.5, 0.5]}/>
<CapsuleCollider args={[0.75, 0.5]} />
</mesh>
</RigidBody>
<group ref={objectInHandRef}>
<Weapon position={[0.3, -0.1, 0.3]} scale={0.3} />
</group>
</>
);
}
Animation of weapon swinging while walking
To make the character's gait more natural, we will add a slight wiggle of the weapon while moving. To create the animation we will use the installed tween.js library.
The Weapon component will be wrapped in a group tag so that you can add a reference to it via the useRef hook.

Let's add some useState to save the animation.

Let's create a function to initialise the animation.

Code Explanation:
const twSwayingAnimation = new TWEEN.Tween(currentPosition) ...
Creating an animation of an object "swinging" from its current position to a new position.const twSwayingBackAnimation = new TWEEN.Tween(currentPosition) ...
Creating an animation of the object returning back to its starting position after the first animation has completed.twSwayingAnimation.chain(twSwayingBackAnimation);
Connecting two animations so that when the first animation completes, the second animation automatically starts.
In useEffect we call the animation initialisation function.

Now it is necessary to determine the moment during which the movement occurs. This can be done by determining the current vector of the character's direction.
If character movement occurs, we will refresh the animation and run it again when finished.

Code Explanation:
const isMoving = direction.length() > 0;
Here the object's movement state is
checked. If the direction vector has a length greater than 0, it means that the object has a direction of movement.if (isMoving && isSwayingAnimationFinished) { ... }
This state is executed if the object is moving and the "swinging" animation has finished.
Player.jsx
import * as THREE from "three";
import * as RAPIER from "@dimforge/rapier3d-compat"
import * as TWEEN from "@tweenjs/tween.js";
import {CapsuleCollider, RigidBody, useRapier} from "@react-three/rapier";
import {useEffect, useRef, useState} from "react";
import {usePersonControls} from "./hooks.js";
import {useFrame} from "@react-three/fiber";
import {Weapon} from "./Weapon.jsx";
const MOVE_SPEED = 5;
const direction = new THREE.Vector3();
const frontVector = new THREE.Vector3();
const sideVector = new THREE.Vector3();
const rotation = new THREE.Vector3();
export const Player = () => {
const playerRef = useRef();
const { forward, backward, left, right, jump } = usePersonControls();
const objectInHandRef = useRef();
const swayingObjectRef = useRef();
const [swayingAnimation, setSwayingAnimation] = useState(null);
const [swayingBackAnimation, setSwayingBackAnimation] = useState(null);
const [isSwayingAnimationFinished, setIsSwayingAnimationFinished] = useState(true);
const rapier = useRapier();
useFrame((state) => {
if (!playerRef.current) return;
// moving player
const velocity = playerRef.current.linvel();
frontVector.set(0, 0, backward - forward);
sideVector.set(left - right, 0, 0);
direction.subVectors(frontVector, sideVector).normalize().multiplyScalar(MOVE_SPEED).applyEuler(state.camera.rotation);
playerRef.current.wakeUp();
playerRef.current.setLinvel({ x: direction.x, y: velocity.y, z: direction.z });
// jumping
const world = rapier.world;
const ray = world.castRay(new RAPIER.Ray(playerRef.current.translation(), { x: 0, y: -1, z: 0 }));
const grounded = ray && ray.collider && Math.abs(ray.toi) <= 1.5;
if (jump && grounded) doJump();
// moving camera
const {x, y, z} = playerRef.current.translation();
state.camera.position.set(x, y, z);
// moving object in hand for the player
objectInHandRef.current.rotation.copy(state.camera.rotation);
objectInHandRef.current.position.copy(state.camera.position).add(state.camera.getWorldDirection(rotation));
const isMoving = direction.length() > 0;
if (isMoving && isSwayingAnimationFinished) {
setIsSwayingAnimationFinished(false);
swayingAnimation.start();
}
TWEEN.update();
});
const doJump = () => {
playerRef.current.setLinvel({x: 0, y: 8, z: 0});
}
const initSwayingObjectAnimation = () => {
const currentPosition = new THREE.Vector3(0, 0, 0);
const initialPosition = new THREE.Vector3(0, 0, 0);
const newPosition = new THREE.Vector3(-0.05, 0, 0);
const animationDuration = 300;
const easing = TWEEN.Easing.Quadratic.Out;
const twSwayingAnimation = new TWEEN.Tween(currentPosition)
.to(newPosition, animationDuration)
.easing(easing)
.onUpdate(() => {
swayingObjectRef.current.position.copy(currentPosition);
});
const twSwayingBackAnimation = new TWEEN.Tween(currentPosition)
.to(initialPosition, animationDuration)
.easing(easing)
.onUpdate(() => {
swayingObjectRef.current.position.copy(currentPosition);
})
.onComplete(() => {
setIsSwayingAnimationFinished(true);
});
twSwayingAnimation.chain(twSwayingBackAnimation);
setSwayingAnimation(twSwayingAnimation);
setSwayingBackAnimation(twSwayingBackAnimation);
}
useEffect(() => {
initSwayingObjectAnimation();
}, []);
return (
<>
<RigidBody colliders={false} mass={1} ref={playerRef} lockRotations>
<mesh castShadow>
<capsuleGeometry args={[0.5, 0.5]}/>
<CapsuleCollider args={[0.75, 0.5]} />
</mesh>
</RigidBody>
<group ref={objectInHandRef}>
<group ref={swayingObjectRef}>
<Weapon position={[0.3, -0.1, 0.3]} scale={0.3} />
</group>
</group>
</>
);
}
In the App component, let's add a useFrame where we will update the tween animation.

TWEEN.update() updates all active animations in the TWEEN.js library. This method is called on each animation frame to ensure that all animations run smoothly.
Section code
Recoil animation
We need to define the moment when a shot is fired - that is, when the mouse button is pressed. Let's add useState to store this state, useRef to store a reference to the weapon object, and two event handlers for pressing and releasing the mouse button.



Let's implement a recoil animation when clicking the mouse button. We will use tween.js library for this purpose.
Let us define constants for recoil force and animation duration.

As with the weapon wiggle animation, we add two useState states for the recoil and return to home position animation and a state with the animation end status.

Let's make functions to get a random vector of recoil animation - generateRecoilOffset and generateNewPositionOfRecoil.

Create a function to initialise the recoil animation. We will also add useEffect, in which we will specify the "shot" state as a dependency, so that at each shot the animation is initialised again and new end coordinates are generated.


And in useFrame, let's add a check for "holding" the mouse key for firing, so that the firing animation doesn't stop until the key is released.

Weapon.jsx
import * as THREE from "three";
import * as TWEEN from "@tweenjs/tween.js";
import {WeaponModel} from "./WeaponModel.jsx";
import {useEffect, useRef, useState} from "react";
import {useFrame} from "@react-three/fiber";
const recoilAmount = 0.03;
const recoilDuration = 100;
const easing = TWEEN.Easing.Quadratic.Out;
export const Weapon = (props) => {
const [recoilAnimation, setRecoilAnimation] = useState(null);
const [recoilBackAnimation, setRecoilBackAnimation] = useState(null);
const [isShooting, setIsShooting] = useState(false);
const weaponRef = useRef();
useEffect(() => {
document.addEventListener('mousedown', () => {
setIsShooting(true);
});
document.addEventListener('mouseup', () => {
setIsShooting(false);
});
}, []);
const generateRecoilOffset = () => {
return new THREE.Vector3(
Math.random() * recoilAmount,
Math.random() * recoilAmount,
Math.random() * recoilAmount,
)
}
const generateNewPositionOfRecoil = (currentPosition) => {
const recoilOffset = generateRecoilOffset();
return currentPosition.clone().add(recoilOffset);
}
const initRecoilAnimation = () => {
const currentPosition = new THREE.Vector3(0, 0, 0);
const initialPosition = new THREE.Vector3(0, 0, 0);
const newPosition = generateNewPositionOfRecoil(currentPosition);
const twRecoilAnimation = new TWEEN.Tween(currentPosition)
.to(newPosition, recoilDuration)
.easing(easing)
.onUpdate(() => {
weaponRef.current.position.copy(currentPosition);
});
const twRecoilBackAnimation = new TWEEN.Tween(currentPosition)
.to(initialPosition, recoilDuration)
.easing(easing)
.onUpdate(() => {
weaponRef.current.position.copy(currentPosition);
});
twRecoilAnimation.chain(twRecoilBackAnimation);
setRecoilAnimation(twRecoilAnimation);
setRecoilBackAnimation(twRecoilBackAnimation);
}
const startShooting = () => {
recoilAnimation.start();
}
useEffect(() => {
initRecoilAnimation();
if (isShooting) {
startShooting();
}
}, [isShooting]);
useFrame(() => {
TWEEN.update();
if (isShooting) {
startShooting();
}
});
return (
<group {...props}>
<group ref={weaponRef}>
<WeaponModel />
</group>
</group>
);
}
Animation during inactivity
Realise the animation of "inactivity" for the character, so that there is no feeling of the game "hanging".
To do this, let's add some new states via useState.

Let's fix the initialisation of the "wiggle" animation to use values from the state. The idea is that different states: walking or stopping, will use different values for the animation and each time the animation will be initialised first.

Player.jsx
import * as THREE from "three";
import * as RAPIER from "@dimforge/rapier3d-compat"
import * as TWEEN from "@tweenjs/tween.js";
import {CapsuleCollider, RigidBody, useRapier} from "@react-three/rapier";
import {useEffect, useRef, useState} from "react";
import {usePersonControls} from "./hooks.js";
import {useFrame} from "@react-three/fiber";
import {Weapon} from "./Weapon.jsx";
const MOVE_SPEED = 5;
const direction = new THREE.Vector3();
const frontVector = new THREE.Vector3();
const sideVector = new THREE.Vector3();
const rotation = new THREE.Vector3();
export const Player = () => {
const playerRef = useRef();
const { forward, backward, left, right, jump } = usePersonControls();
const objectInHandRef = useRef();
const swayingObjectRef = useRef();
const [swayingAnimation, setSwayingAnimation] = useState(null);
const [swayingBackAnimation, setSwayingBackAnimation] = useState(null);
const [isSwayingAnimationFinished, setIsSwayingAnimationFinished] = useState(true);
const [swayingNewPosition, setSwayingNewPosition] = useState(new THREE.Vector3(-0.005, 0.005, 0));
const [swayingDuration, setSwayingDuration] = useState(1000);
const [isMoving, setIsMoving] = useState(false);
const rapier = useRapier();
useFrame((state) => {
if (!playerRef.current) return;
// moving player
const velocity = playerRef.current.linvel();
frontVector.set(0, 0, backward - forward);
sideVector.set(left - right, 0, 0);
direction.subVectors(frontVector, sideVector).normalize().multiplyScalar(MOVE_SPEED).applyEuler(state.camera.rotation);
playerRef.current.wakeUp();
playerRef.current.setLinvel({ x: direction.x, y: velocity.y, z: direction.z });
// jumping
const world = rapier.world;
const ray = world.castRay(new RAPIER.Ray(playerRef.current.translation(), { x: 0, y: -1, z: 0 }));
const grounded = ray && ray.collider && Math.abs(ray.toi) <= 1.5;
if (jump && grounded) doJump();
// moving camera
const {x, y, z} = playerRef.current.translation();
state.camera.position.set(x, y, z);
// moving object in hand for the player
objectInHandRef.current.rotation.copy(state.camera.rotation);
objectInHandRef.current.position.copy(state.camera.position).add(state.camera.getWorldDirection(rotation));
setIsMoving(direction.length() > 0);
if (swayingAnimation && isSwayingAnimationFinished) {
setIsSwayingAnimationFinished(false);
swayingAnimation.start();
}
});
const doJump = () => {
playerRef.current.setLinvel({x: 0, y: 8, z: 0});
}
const setAnimationParams = () => {
if (!swayingAnimation) return;
swayingAnimation.stop();
setIsSwayingAnimationFinished(true);
if (isMoving) {
setSwayingDuration(() => 300);
setSwayingNewPosition(() => new THREE.Vector3(-0.05, 0, 0));
} else {
setSwayingDuration(() => 1000);
setSwayingNewPosition(() => new THREE.Vector3(-0.01, 0, 0));
}
}
const initSwayingObjectAnimation = () => {
const currentPosition = new THREE.Vector3(0, 0, 0);
const initialPosition = new THREE.Vector3(0, 0, 0);
const newPosition = swayingNewPosition;
const animationDuration = swayingDuration;
const easing = TWEEN.Easing.Quadratic.Out;
const twSwayingAnimation = new TWEEN.Tween(currentPosition)
.to(newPosition, animationDuration)
.easing(easing)
.onUpdate(() => {
swayingObjectRef.current.position.copy(currentPosition);
});
const twSwayingBackAnimation = new TWEEN.Tween(currentPosition)
.to(initialPosition, animationDuration)
.easing(easing)
.onUpdate(() => {
swayingObjectRef.current.position.copy(currentPosition);
})
.onComplete(() => {
setIsSwayingAnimationFinished(true);
});
twSwayingAnimation.chain(twSwayingBackAnimation);
setSwayingAnimation(twSwayingAnimation);
setSwayingBackAnimation(twSwayingBackAnimation);
}
useEffect(() => {
setAnimationParams();
}, [isMoving]);
useEffect(() => {
initSwayingObjectAnimation();
}, [swayingNewPosition, swayingDuration]);
return (
<>
<RigidBody colliders={false} mass={1} ref={playerRef} lockRotations>
<mesh castShadow>
<capsuleGeometry args={[0.5, 0.5]}/>
<CapsuleCollider args={[0.75, 0.5]} />
</mesh>
</RigidBody>
<group ref={objectInHandRef}>
<group ref={swayingObjectRef}>
<Weapon position={[0.3, -0.1, 0.3]} scale={0.3} />
</group>
</group>
</>
);
}
Conclusion
In this part we have implemented scene generation and character movement. We also added a weapon model, recoil animation when firing and at idle. In the next part we will continue to refine our game, adding new functionality.